Title: Building a “Resume Rizz” App with FastAPI, Azure Form Recognizer, and OpenAI
If you’ve ever wanted to combine the power of AI resume analysis with a simple payment flow, you’ll enjoy this little project we’re calling “Resume Rizz.” In this post, I’ll walk you through the high-level components of the app, why I built it, and how it works under the hood. If you’d like to check it out, head on over to resumerizz.io!
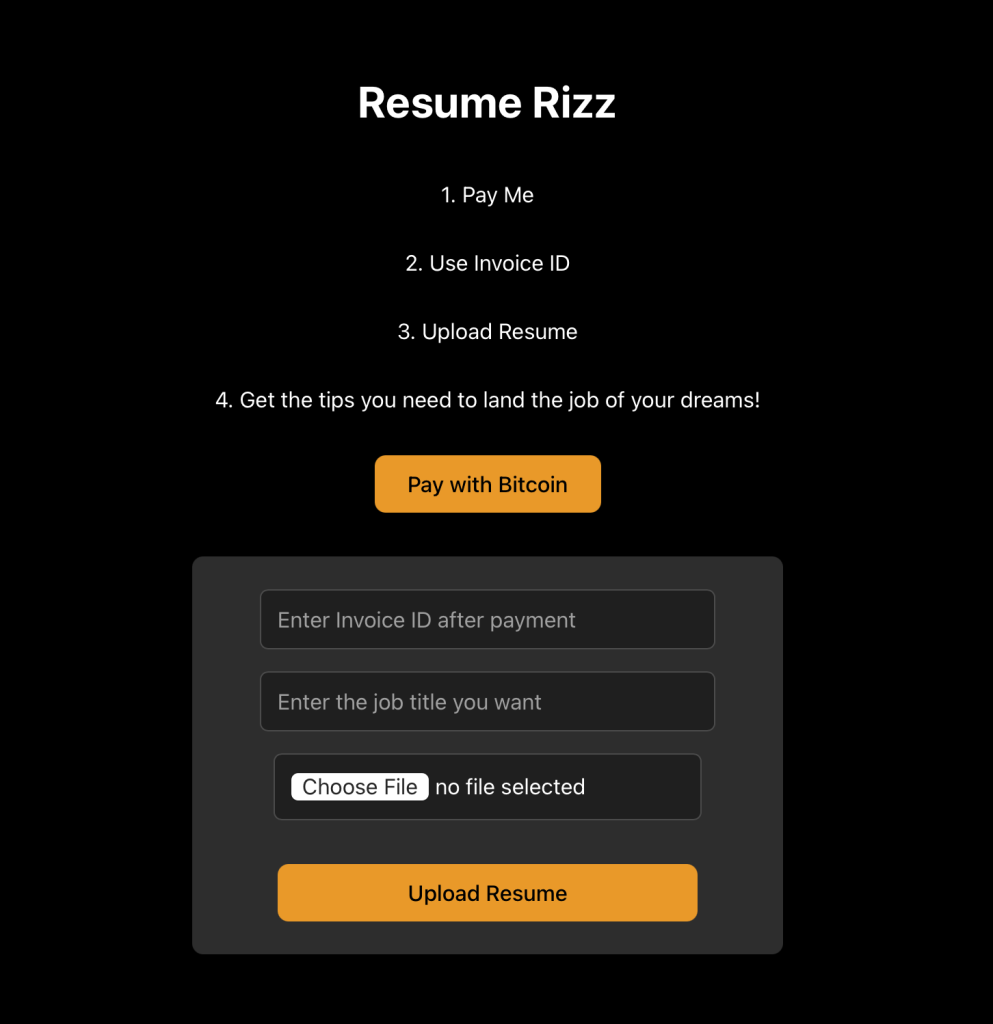
1. What Does It Do?
We all know the job market can be competitive. “Resume Rizz” aims to give your resume that extra spark—or “rizz”—by offering targeted feedback and suggestions. With a quick payment, you can upload your resume, and the app will:
- Check your payment via BTCPay Server.
- Analyze your resume with Azure Form Recognizer to extract text.
- Use OpenAI to provide personalized feedback based on your desired job title.
Key Tech Stack:
- FastAPI (Python) for the backend.
- Azure Form Recognizer to parse resumes.
- OpenAI for GPT-based feedback.
- BTCPay for Bitcoin invoice creation and payment checks.
- React (JavaScript) for the frontend.
- Azure App Service to host the backend
- Azure Static Apps to host the frontend
2. Overview of the Architecture
Here’s the basic flow:
- User pays via Bitcoin using a generated invoice from the backend.
- User receives an invoice ID to confirm payment.
- User uploads their resume alongside that invoice ID and the job title they’re interested in.
- Backend checks payment with BTCPay, then uses Form Recognizer to extract text from the resume PDF or DOCX file.
- OpenAI analyzes the extracted text and returns helpful feedback on how to improve the resume for that specific job title.
- Frontend displays the analysis results in a neat, user-friendly format.
3. FastAPI Backend Highlights
We used FastAPI for its simplicity and speed:
/create-btc-invoice/
: Generates a BTC invoice using BTCPay./check-btc-payment/
: Confirms if an invoice is settled./upload-resume/
:- Validates invoice payment.
- Sends the resume to Azure Form Recognizer.
- Sends the recognized text to OpenAI’s GPT model.
- Returns the feedback to the user.
All environment variables (keys, tokens, URLs) are stored in a .env
file and loaded securely. This approach ensures we never commit secrets to our repository.
4. Using Azure Form Recognizer
Azure Form Recognizer helps parse PDF or DOCX files into raw text. The relevant steps are:
- Read the uploaded file in FastAPI.
- Send the file to
DocumentAnalysisClient
with theprebuilt-document
model. - Extract the lines of text from the recognized structure.
- Join them into a single string to send to OpenAI.
This means you don’t have to manually parse PDFs or DOCX—Form Recognizer handles it for you.
5. GPT-4o for Feedback
Once we have the extracted text:
- OpenAI is called with a prompt that includes the user’s job title and the recognized text.
- We use
model="gpt-4o"
to generate constructive feedback. - The GPT response is then returned to the frontend.
It’s amazing how quickly GPT can provide pointed advice on how to structure your resume, highlight relevant skills, and even note small improvements you might not have considered.
6. The React Frontend
The frontend is a straightforward React app that:
- Shows a “Pay with Bitcoin” button, which calls the backend to create an invoice.
- Accepts Invoice ID, Job Title, and File Upload.
- Posts these fields to the backend’s
/upload-resume/
endpoint. - Displays the analysis in a user-friendly way once the response comes back.
We also added a dark theme with black backgrounds, gold buttons, and white text for a modern look.
7. Payment with BTCPay
BTCPay is an open-source Bitcoin payment processor:
- When a user clicks “Pay with Bitcoin”, the backend calls BTCPay to generate an invoice.
- The user is redirected to the BTCPay checkout page.
- The invoice ID is stored, so once the user enters it in the resume upload form, we can check if it’s “Settled.”
- If it’s not settled, we reject the resume upload with a
400
error. If it’s confirmed, we proceed to the AI analysis.
This ensures we only run AI analysis for paid invoices.
8. Lessons Learned
- Form Recognizer can drastically simplify PDF parsing.
- OpenAI is extremely flexible for generating feedback, but watch out for API usage and model access.
- BTCPay is a powerful way to handle BTC payments, but you need to handle invoice confirmations and statuses carefully.
- FastAPI remains a great framework for quickly building secure, high-performance microservices.
9. Possible Future Improvements
- Additional Payment Options: Right now, we only accept BTC, but you could integrate credit cards or other crypto.
- Resume Storage: If you want to store resumes or keep logs for a portfolio of feedback, consider Azure Blob Storage or a database.
- Enhanced Resume Parsing: You could use custom Form Recognizer models or parse additional fields (like name, address, etc.).
- More AI Models: Expand the analysis to other GPT-based endpoints or incorporate specialized job-lingo suggestions.
Conclusion
“Resume Rizz” is a fun project that shows how to integrate various cloud services—Azure Form Recognizer for parsing, OpenAI for AI feedback, BTCPay for crypto payments, and FastAPI for orchestrating everything. The final product lets users quickly pay, upload a resume, and get targeted advice in a single workflow.
If you’re curious to see the code or try it out, feel free to reach out!
Happy Coding!